Time Space Complexity Cheat Sheet
Java collections time complexity
Java Collections – Performance (Time Complexity) Many developers I came across in my career as a software developer are only familiar with the most basic data structures, typically, Array, Map and Linked List. These are fundamental data structures and one could argue that they are generic enough to fit most of the commercial software requirements.
Know Thy Complexities! This webpage covers the space and time Big-O complexities of common algorithms used in Computer Science. When preparing for technical interviews in the past, I found myself spending hours crawling the internet putting together the best, average, and worst case complexities for search and sorting algorithms so that I wouldn't be stumped when asked about them. O(n2)O(n^2)O(n2) O(1)O(1)O(1) Selection sort works by repeatedly 'selecting' the.
1. The time complexity of Collections.sort () is O (n*log (n)) and a list sorted with Collections.sort () will only be sorted after the call to sort () Info present in collections docs - 'The sorting algorithm is a modified mergesort (in which the merge is omitted if the highest element in the low sublist is less than the lowest element in the high sublist).
Runtime Complexity of Java Collections. GitHub Gist: instantly share code, notes, and snippets.
Arraylist time complexity
ArrayList vs. LinkedList vs. Vector, for arbitrary indices of add/remove, but O(1) for operations at end/beginning of the List. The arraylist is basically an implementation of array. so the time complexity of the CRUD operations on it would be : get/read : O(1) since you can seek the address directly from base remove/delete : O(n) why ? Because once we delete the element at index, then we need to move the after values one by one to the left.

Time Complexity of Java Collections, An ArrayList in Java is a List that is backed by an array . The get(index) method is a constant time, O(1) , operation. The code straight out of the Time Complexity for Java ArrayList. Ask Question Asked 9 years, 1 month ago. Active 6 years, 2 months ago. Viewed 53k times 27. 4. I found other entries for this
Time complexity for java ArrayList, Both have time complexity O(1), but due to the added steps of creating a new array in ArrayList its worst-case complexity reaches to order of N, The second point is that that the complexity of ArrayList.remove (index) is sensitive to the value of index as well as the list length. The 'advertised' complexity of O (N) for the average and worst cases. In the best case, the complexity is actually O (1).
Space complexity

What does 'Space Complexity' mean?, Space Complexity of an algorithm is total space taken by the algorithm with respect to the input size. Space complexity includes both Auxiliary The space complexity of an algorithm or a computer program is the amount of memory space required to solve an instance of the computational problem as a function of characteristics of the input. It is the memory required by an algorithm to execute a program and produce output.
EECS 311: Space Complexity, Space complexity is a measure of the amount of working storage an algorithm needs. That means how much memory, in the worst case, is needed at any point in Space complexity in algorithm development is a metric for how much storage space the algorithm needs in relation to its inputs. This measurement is extremely useful in some kinds of programming evaluations as engineers, coders and other scientists look at how a particular algorithm works. Techopedia explains Space Complexity
Space complexity, The space complexity of an algorithm or a computer program is the amount of memory space required to solve an instance of the computational problem as a Space complexity is an amount of memory used by the algorithm (including the input values of the algorithm), to execute it completely and produce the result. We know that to execute an algorithm it must be loaded in the main memory. The memory can be used in different forms: Variables (This includes the constant values and temporary values)
Time complexity cheat sheet
Big-O Algorithm Complexity Cheat Sheet (Know Thy Complexities , This webpage covers the space and time Big-O complexities of common algorithms used in Computer Science. When preparing for technical interviews in the Know Thy Complexities! Hi there! This webpage covers the space and time Big-O complexities of common algorithms used in Computer Science. When preparing for technical interviews in the past, I found myself spending hours crawling the internet putting together the best, average, and worst case complexities for search and sorting algorithms so that I wouldn't be stumped when asked about them.
[PDF] Big-O Algorithm Complexity Cheat Sheet, Data Structure. Time Complexity. Space Complexity. Average. Worst. Worst. Access Search Insertion Deletion Access Search Insertion Deletion. Array. Θ(1). Data Structure Time Complexity Space Complexity Average Worst Worst Big-O Algorithm Complexity Cheat Sheet Created Date: 7/10/2016 7:49:20 PM
Big o Cheatsheet, Big o cheatsheet with complexities chart Big o complete Graph ![Bigo graph][1] Legend ![legend][3] ![Big o Algorithms with thier complexities. Time-complexity. Time complexity Cheat Sheet. BigO Graph *Correction:- Best time complexity for TIM SORT is O(nlogn) Tweet. Author. Vipin Khushu. Software Development Engineer at Amazon.
Time complexity examples pdf
[PDF] Time complexity, have a complexity of O(n), even though the running time of the program may vary. When analyzing the complexity we must look for specific, worst-case examples complexity instead of worrying about a faster solution. For example, if: • n 1000000, the expected time complexity is O(n) or O(nlogn), • n 10000, the expected time complexity is O(n2), • n 500, the expected time complexity is O(n3). Of course, these limits are not precise. They are just approximations, and will vary depending
[PDF] Time complexity :, O(n) linear time. This means that the algorithm requires a number of steps proportional to the size of the task. Examples: 1. Traversing an array. 2. Sequential/ the dominant term determines the time complexity) O(log n) logarithmic time Examples: 1. Binary search in a sorted array of n elements. O(n log n) 'n log n ' time Examples: 1. MergeSort, QuickSort etc.
[PDF] 1 Exercises and Solutions, An algorithm with time complexity O(f(n)) and processing time T(n) = cf(n), where f(n) is a known function of n, spends 10 seconds to process. 1000 data items. How Algorithm complexity • The Big-O notation: – the running time of an algorithm as a function of the size of its input – worst case estimate – asymptotic behavior • O(n2) means that the running time of the algorithm on an input of size n is limited by the quadratic function of n 8
Time complexity of array
Data Structures and Algorithms/Arrays, Lists and Vectors, Data Structure, Time Complexity, Space Complexity. Average, Worst, Worst. Access, Search, Insertion, Deletion, Access, Search, Insertion, Deletion. Array, Θ(1) Time complexity analysis . Using the index value, we can access the array elements in constant time. So the time complexity is O(1) for accessing an element in the array.
Time complexity of array/list operations [Java, Python] · YourBasic, This can lead to a problem of time complexity. Because it takes a single step to access an item of an array via its index, or add/remove an The compile-time complexity is O(1) for each variable (it just needs to look up the size of the datatype, and multiply by the size if it's an array), so O(n) where n is the number of local variables. share|improve this answer|follow |. edited May 2 '16 at 21:58.
Big-O Algorithm Complexity Cheat Sheet (Know Thy Complexities , Arrays takes O(1) time to access an element. Linked list takes O(1) if pointer is given,otherwise O(n). · Deletion in arrays takes O(n) while linked lists takes O(1) if It depends how the array is represented. If it's just a contiguous string of memory locations, it might require [math]O(n)[/math] work, as every following element must be shifted down or up one place, or, if the memory following it can't be reallo
Algorithm complexity
Algorithmic complexity is concerned about how fast or slow particular algorithm performs. We define complexity as a numerical function T(n)- time versus the input size n. We want to define time taken by an algorithm without depending on the implementation details. But you agree that T(n)does depend on the implementation!
Time And Space Complexity Cheat Sheet
Algorithmic complexity is a measure of how long an algorithm would take to complete given an input of size n. If an algorithm has to scale, it should compute the result within a finite and practical time bound even for large values of n. For this reason, complexity is calculated asymptotically as n approaches infinity.
The (computational) complexity of an algorithm is a measure of the amount of computing resources (time and space) that a particular algorithm consumes when it runs. Visual studio code for python tutorial. Computer scientists use mathematical measures of complexity that allow them to predict, before writing the code, how fast an algorithm will run and how much memory it will require.
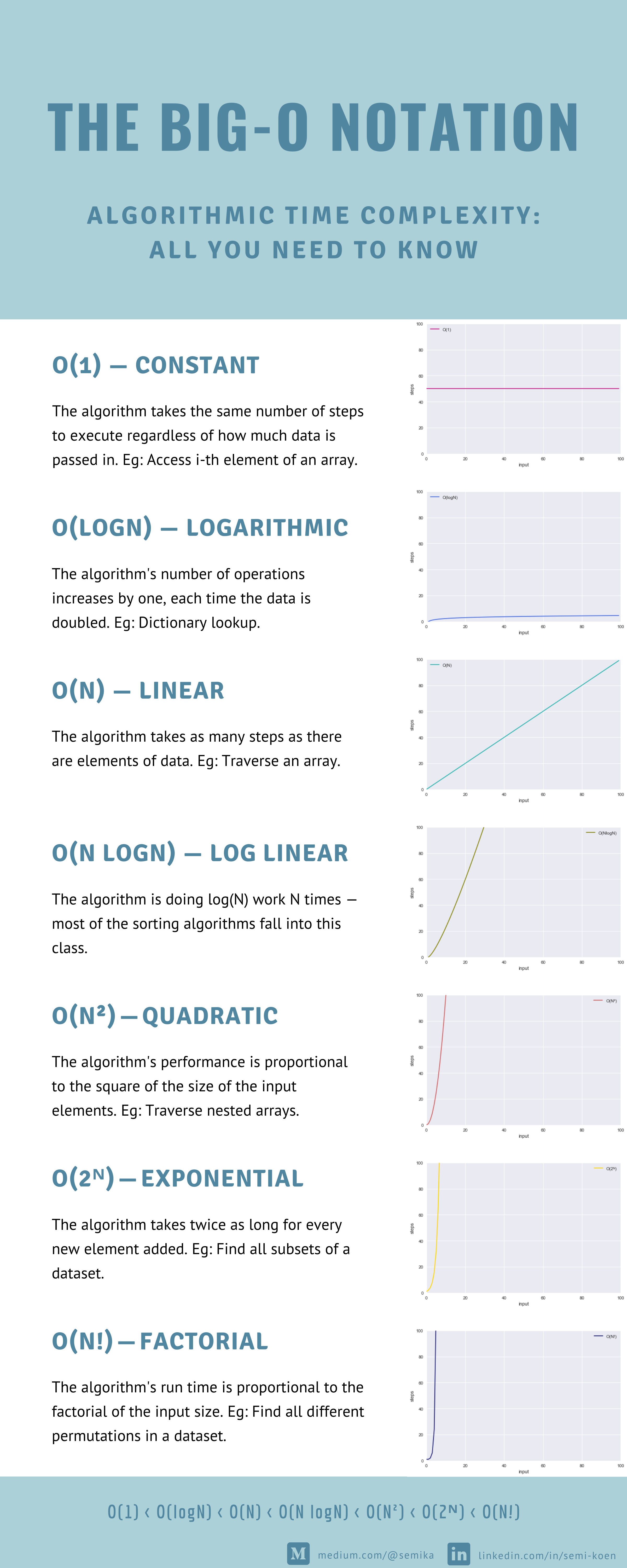
Java hashmap time complexity
On an average the time complexity of a HashMap insertion, deletion, the search takes O(1) constant time. That said, in the worst case, java takes O(n) time for searching, insertion, and deletion. Mind you, the time complexity of HashMap apparently depends on the loadfactor n/b (the number of entries present in the hash table BY the total number of buckets in the hashtable) and how efficiently the hash
Time Space Complexity Cheat Sheet
Hashmap put and get operation time complexity is O(1) with assumption that key-value pairs are well distributed across the buckets. It means hashcode implemented is good. In above Letter Box example, If say hashcode() method is poorly implemented and returns hashcode 'E' always, In this case.
The time complexity of containsKey has changed in JDK-1.8, as others mentioned it is O(1) in ideal cases. However, in case of collisions where the keys are Comparable, bins storing collide elements aren't linear anymore after they exceed some threshold called TREEIFY_THRESHOLD, which is equal to 8,

Cheat Sheet For Time Sheets
More Articles
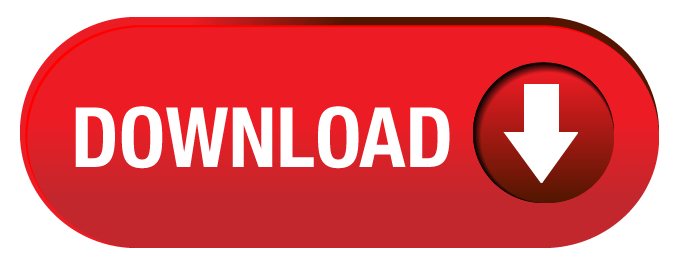