Solidity Cheat Sheet
- Solidity Cheat Sheet Download
- Solidity Cheat Sheet 2019
- Solidity Cheat Sheet
- Solidity Cheat Sheets
- Solidity Cheat Sheet Pdf
super.function();
- call func from first ancestorEatTheBlocks Solidity Video Tutorials; Quickblocks; LearnChannels; VDF Research; Awesome Cryptoeconomics; Web3 Foundation Wiki; Zero-Knowledge Proofs Starter Pack; Awesome Ethreum Virtual Machine; Solidity and Vyper cheat sheet; Solidity Development Guides; Security. MyCrypto's Security Guide; Hardware Wallet Guide; Paper Wallet Guide; Common. Suited Up Code is a web design and development blog that publishes articles and tutorials about the latest web trends, techniques and new possibilities offered by the front end technologies (HTML, CSS, JavasScript, JQuery, Angular etc etc).
Do not rely on block.timestamp or blockhash as a source of randomness, unless you know what you are doing. Both the timestamp and the block hash can be influenced by miners to some degree. Bad actors in the mining community can for example run a casino payout function on a chosen hash and just retry a different hash if they did not receive any money. The following links provide an overview for a developer interested in getting started with Ethereum. (Of course, this is not exhaustive) Tooling & Frameworks Solidity Browser Remix Tool Eth Stats Metamask Infura for Ethereum and IPFS My Command Cheatsheet Ethereum Testnet Evolution of Ethereum Testnet Specifications ERC: Ethereum Smart Contract Packaging Specification Ethereum Package. From Solidity Documentation Tips and Tricks. Use delete on arrays to delete all its elements. Delete paidPlayers; This is exactly the same as paidPlayers.length = 0. Improve this answer. Follow answered Apr 20 '18 at 3:35.
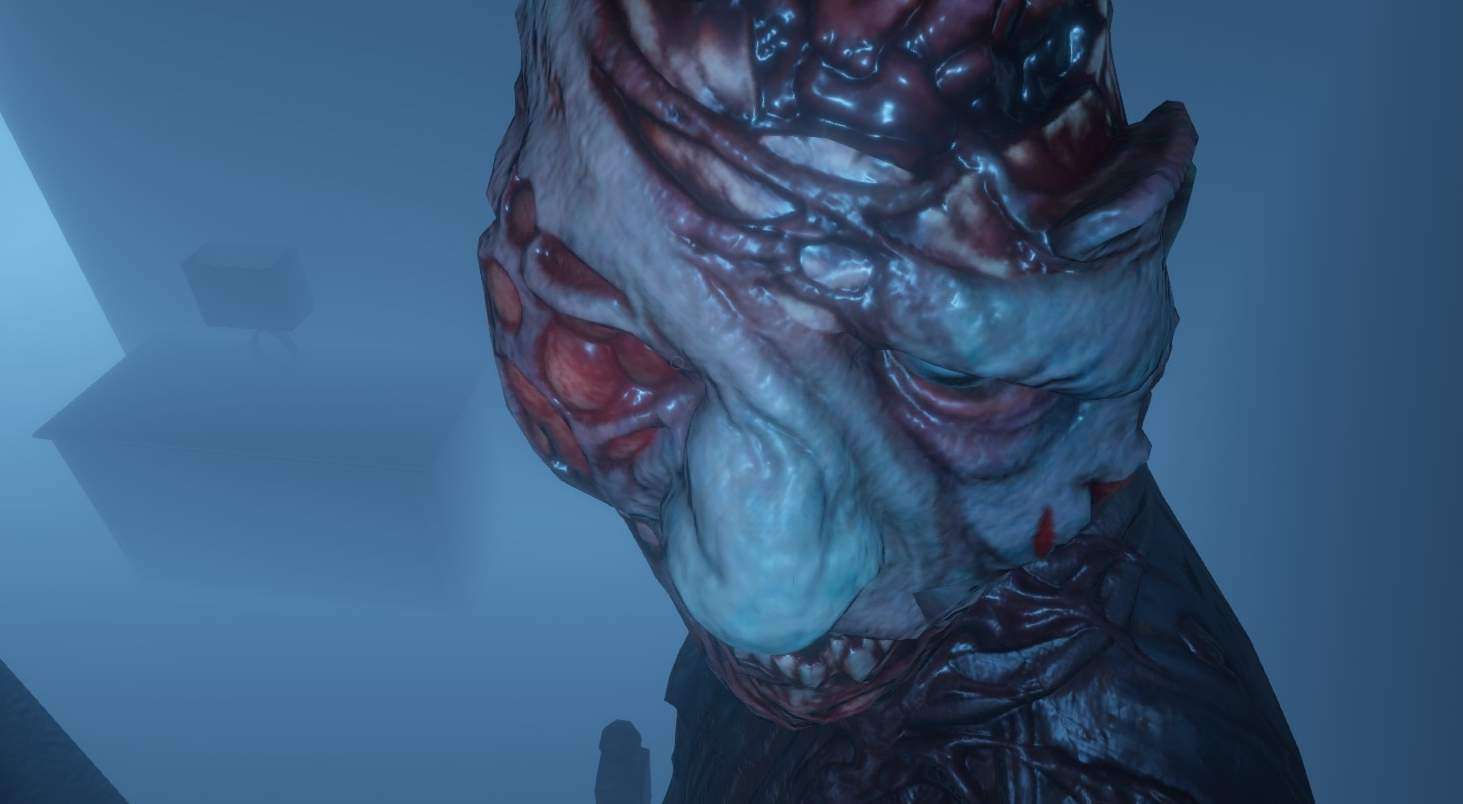
SpecificAncestor.function();
- call func from specific ancestorAncestor contructors
contract Derived is Base(7) { .. };
- pass a static value to ancestor constructorconstructor(uint _y) Base(_y * _y) public {};
- pass a dynamic valueAll ancestor constructors are called upon contract initialization and all needs to have theirs arguments set in one of the ways above.
require / revert / assert
require(condition,[message])
- use for public facing assertionsrevert([message])
assert(condition)
- use for internal assertionsAll of these throw an error and revert current transaction.
Inline assembly
assembly { .. }
- block with inline assembly to get closer to EVM Deprecations
constant
- function modifieryear
- time literal suffixthrow
- alias to revertblock.blockhash(block_number);
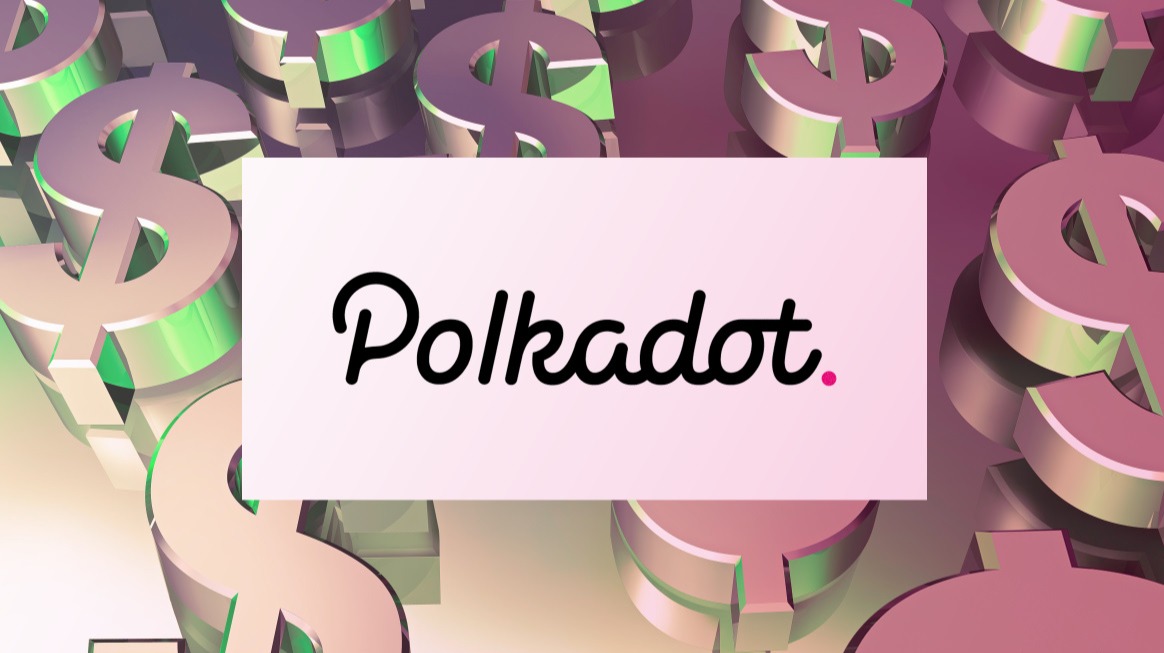
blockhash(block_number);
msg.gas
- replaced by global gasleft();
suicide
- alias to selfdestruct(address)
keccak256(a, b)
- deprecated in favor ofkeccak256(abi.encodePacked(a, b))
callcode
One of the easiest ways to build, deploy, and test solidity code is by using the:
- Metamask wallet.
To get started, download the Metamask Browser Extension.
Once installed, we will be working with Remix. The below code will be pre-loaded, but before we head over there, let’s look at a few tips to get started with remix. Load it all by hitting this link.
- Choose the Solidity compiler
- Open the file loaded by that link
- Compile the file
- Deploy
- Play with contracts
You’ve deployed your first contract! Congrats!

You can test out and play with the functions defined. Check out the comments to learn about what each does.
Working on a testnet
Deploying and testing on a testnet is the most accurate way to test your smart contracts in solidity. To do this let’s first get some testnet ETH from the Kovan testnet. Diagram templates for mac.
Build craft for mac. Pop into this Gitter Channel and drop your metamask address in.
In your metamask, you’ll want to change to the Kovan
testnet.
You’ll be given some free test Ethereum. Ethereum is needed to deploy smart contracts when working with a testnet.
In the previous example, we didn’t use a testnet, we deployed to a fake virtual environment. When working with a testnet, we can actually see and interact with our contracts in a persistent manner.
To deploy to a testnet, on the #4 Deploy
step, change your environment
to injected web3
.This will use whatever network is currently selected in your metamask as the network to deploy to.
For now, please continue to use the Javascript VM
unless instructed otherwise. When you deploy to a testnet, metamask will pop up to ask you to “confirm” the transaction. Hit yes, and after a delay, you’ll get the same contract interface at the bottom of your screen. Best air mouse remote 2020.
Work with the full example below using the Javascript VM
in remix here.
Some more functions.
Additional resources
Smart Contract Development Frameworks
Important libraries
- Zeppelin: Libraries that provide common contract patterns (crowdfuding, safemath, etc)
- Chainlink: Code that allows you to interact with external data
Sample contracts
Security
Style
- Solidity Style Guide: Ethereum’s style guide is heavily derived from Python’s PEP 8 style guide.
Editors
- Editor Snippets (Ultisnips format)
Future To Dos
Solidity Cheat Sheet Download
- New keywords: protected, inheritable
- List of common design patterns (throttling, RNG, version upgrade)
- Common security anti patterns
Solidity Cheat Sheet 2019
Feel free to send a pull request with any edits - or email nemild -/at-/ gmail
Solidity Cheat Sheet
Got a suggestion? A correction, perhaps? Open an Issue on the Github Repo, or make a pull request yourself!
Solidity Cheat Sheets

Solidity Cheat Sheet Pdf
Originally contributed by Nemil Dalal, and updated by 18 contributor(s).
